Understanding SBOMs: A Developer's Guid
- Visakh Unni
- Jan 29
- 16 min read
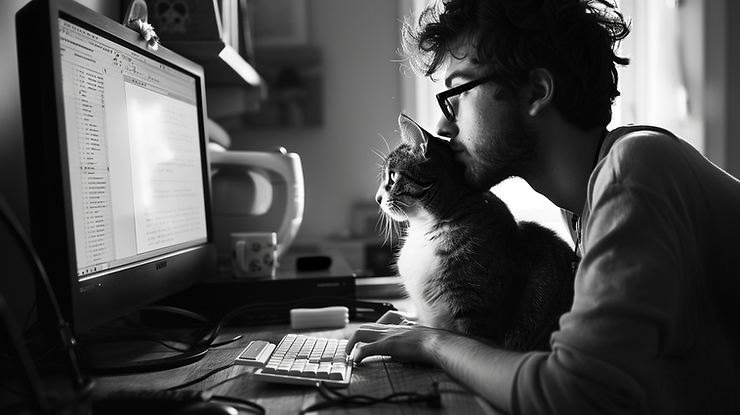
In DevSecOps, the concept of a Software Bill of Materials (SBOM) is gaining traction as a crucial tool for enhancing security, compliance, and quality in our digital solutions. But what exactly is an SBOM, and why should developers care? Let's take a pass at some core concepts involved,
What is an SBOM?
At its foundation, an SBOM functions as a comprehensive inventory for software, detailing every component involved in building a software application. It encompasses the code crafted by the developers themselves as well as all external libraries, frameworks, and dependencies utilized in the software.
The Importance of SBOMs
Enhancing Security
Imagine you're using a third-party library in your application. One day, a severe vulnerability is discovered in that library. How quickly can you determine if your application is at risk? With an SBOM, identifying and addressing such vulnerabilities becomes significantly easier. It's like having a map that shows you exactly where the vulnerable component is within your software's architecture.
Let's say your application uses the requests library for HTTP requests in Python. If a vulnerability is found in requests, you can quickly check your SBOM to see if your application is affected.
Code for generating SBOM:
# Simplified code snippet to generate a list of dependencies (pseudo-code)
dependencies = ['requests==2.25.1', 'numpy==1.21.0', 'pandas==1.3.1']
sbom = {'application': 'MyApp', 'dependencies': dependencies}
print(sbom)
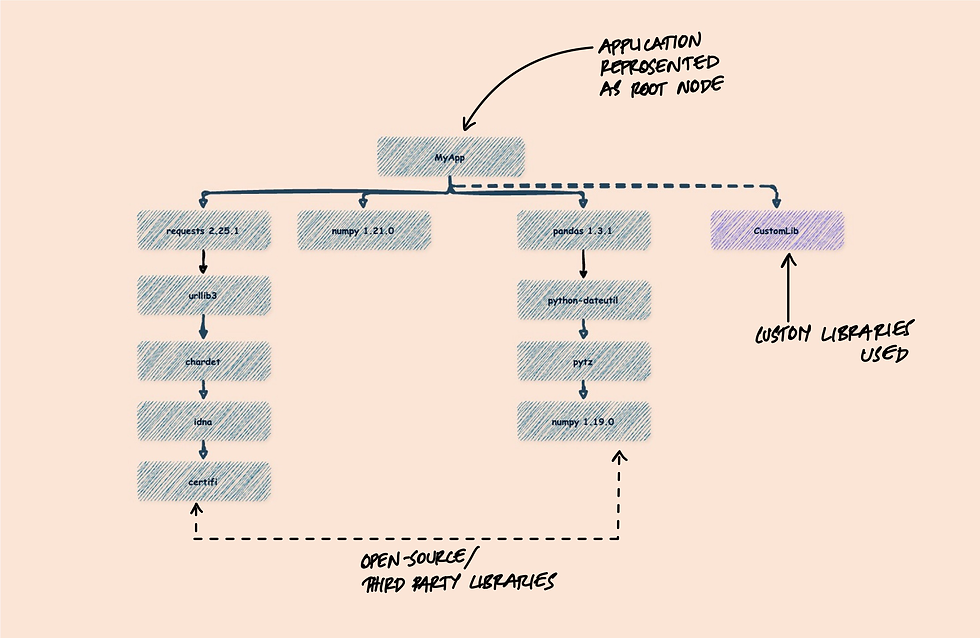
Ensuring Compliance
For developers, ensuring that their software complies with various open-source licenses is paramount. An SBOM can help track the licenses of every component used, making it easier to adhere to legal requirements.
Example: If your application includes a GPL-licensed library, your SBOM will highlight this, allowing you to ensure compliance with the GPL's requirements.

Quality Control and Supply Chain Transparency
An SBOM offers a clear view of the components' quality within your software, ensuring they meet your standards. Additionally, it provides transparency about your software's supply chain, illuminating the origins of each component.
"Using an SBOM for quality control and supply chain transparency in software is like having x-ray vision: you see through layers of code to the origins and quality of every component, ensuring your application is built not just on trust, but on verifiable excellence."
Example: Before integrating a new library, you can consult your SBOM to assess whether it aligns with your quality criteria and doesn't introduce unwanted dependencies.
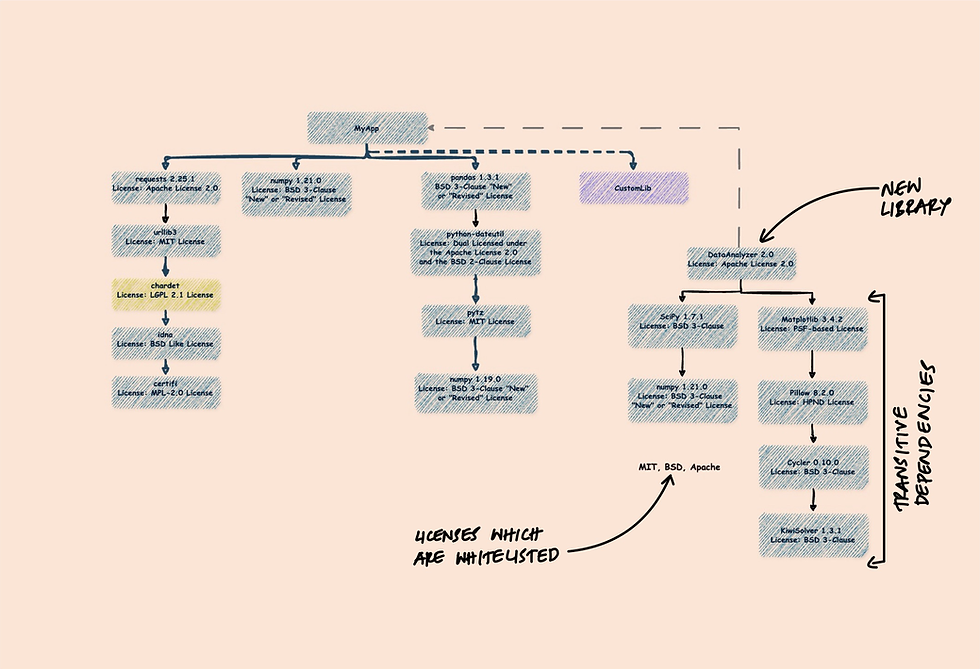
Incident Response
In the event of a security breach, an SBOM can be invaluable for impact analysis and root cause analysis, helping to quickly identify affected components and their dependencies.
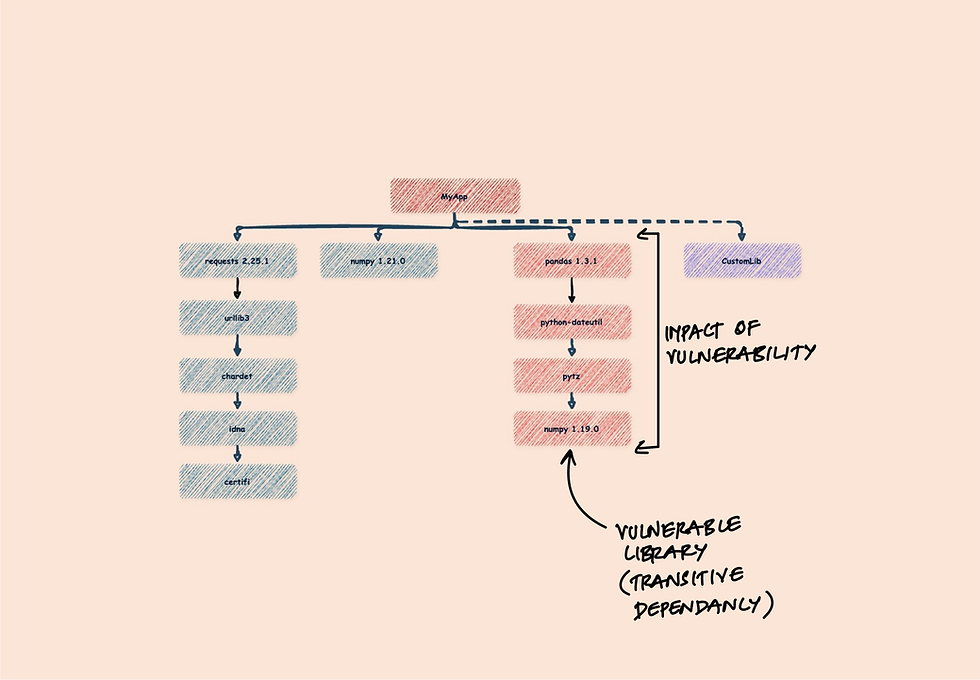
Regulatory and Industry Initiatives
The push for SBOM adoption is not just a grassroots movement within the developer community; it's also being propelled by significant regulatory and industry initiatives. Notably, the U.S. government's executive order on improving the nation's cybersecurity in 2021 underscored the importance of SBOMs for software sold to the U.S. government, spotlighting the role of SBOMs in national security. Similar initiatives are underway in Europe, aiming to bolster software transparency and security on a global scale.
The Log4J Incident: A Wake-up Call
The Log4J incident, a significant security event that underscored the importance of SBOM analysis on a global scale, centered around a vulnerability in Log4J, a popular Java-based logging library open-sourced and widely utilized. Discovered by a member of the Alibaba cloud security team and reported to Apache on November 24, 2021, this vulnerability, identified as CVE-2021-44228 or Log4Shell, was a critical Remote Code Execution (RCE) flaw.
It permitted attackers to run arbitrary code on servers using the affected Log4J version. With a maximum severity score of 10 out of 10 according to the Common Vulnerability Scoring System (CVSS), it highlighted an extreme risk level. The core of the issue lay in Log4J's capability to fetch data from external sources via the Java Naming and Directory Interface (JNDI), which could be manipulated to execute malicious code remotely, demonstrating a profound security threat. This high-severity vulnerability affected countless applications worldwide, illustrating the critical need for SBOMs in identifying and mitigating such threats swiftly.
SBOM Elements: What's Inside?
An SBOM is not just a list; it's a detailed catalog that includes version information, licensing details, and metadata for each software component. This comprehensive inventory aids developers in tracking dependencies, ensuring compliance, and managing security risks effectively.
Example: For an application named DataAnalyzer, an SBOM would detail its dependencies, such as Pandas, NumPy, and Matplotlib, along with their versions, licenses, and other pertinent information.
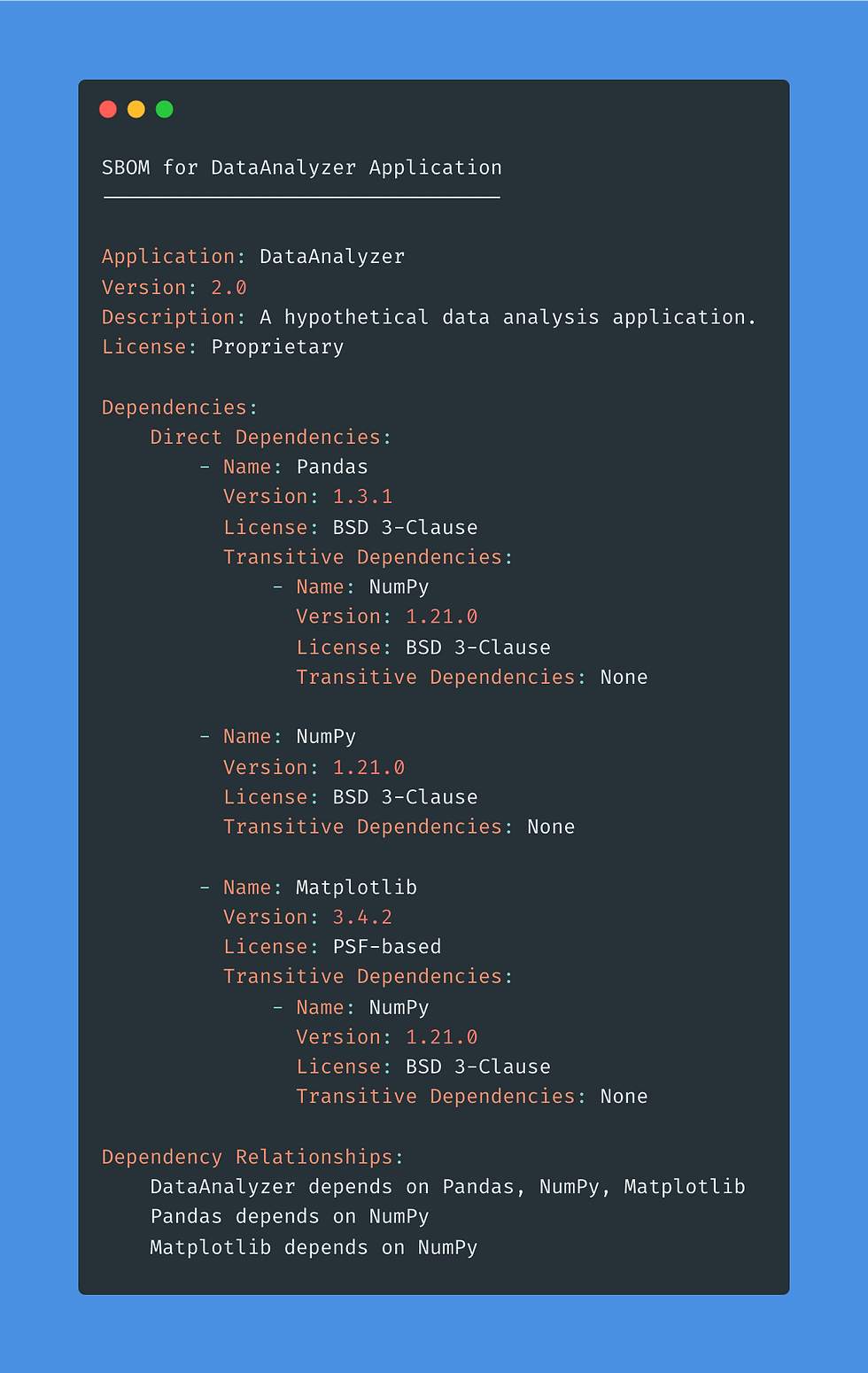
SBOM Standards: Finding Common Ground
To facilitate the creation and exchange of SBOMs, several standards have emerged, with CycloneDX and SPDX being among the most prominent. These standards provide frameworks for documenting software components in a structured, interoperable manner.
Example Scenario: For our DataAnalyzer application, generating an SBOM in both CycloneDX and SPDX formats would ensure comprehensive documentation of its dependencies, such as Pandas, NumPy, and Matplotlib, in a manner that's both readable and machine-processable.
Let's proceed to generate the CycloneDX and SPDX documents for the DataAnalyzer example.
SBOM Creation for DataAnalyzer: A Practical Walkthrough
In our exploration of Software Bill of Materials (SBOMs), we've illustrated their significance with a practical example: the DataAnalyzer application. This Python-based tool is designed for sophisticated data analysis and visualization, relying on several dependencies like Pandas, NumPy, and Matplotlib. Now, let's delve into how we can document these dependencies using SBOM standards, specifically CycloneDX and SPDX.
CycloneDX Example for DataAnalyzer
CycloneDX is a lightweight SBOM standard designed for use in application security contexts and supply chain component analysis. Here's a simplified CycloneDX SBOM for our DataAnalyzer application:
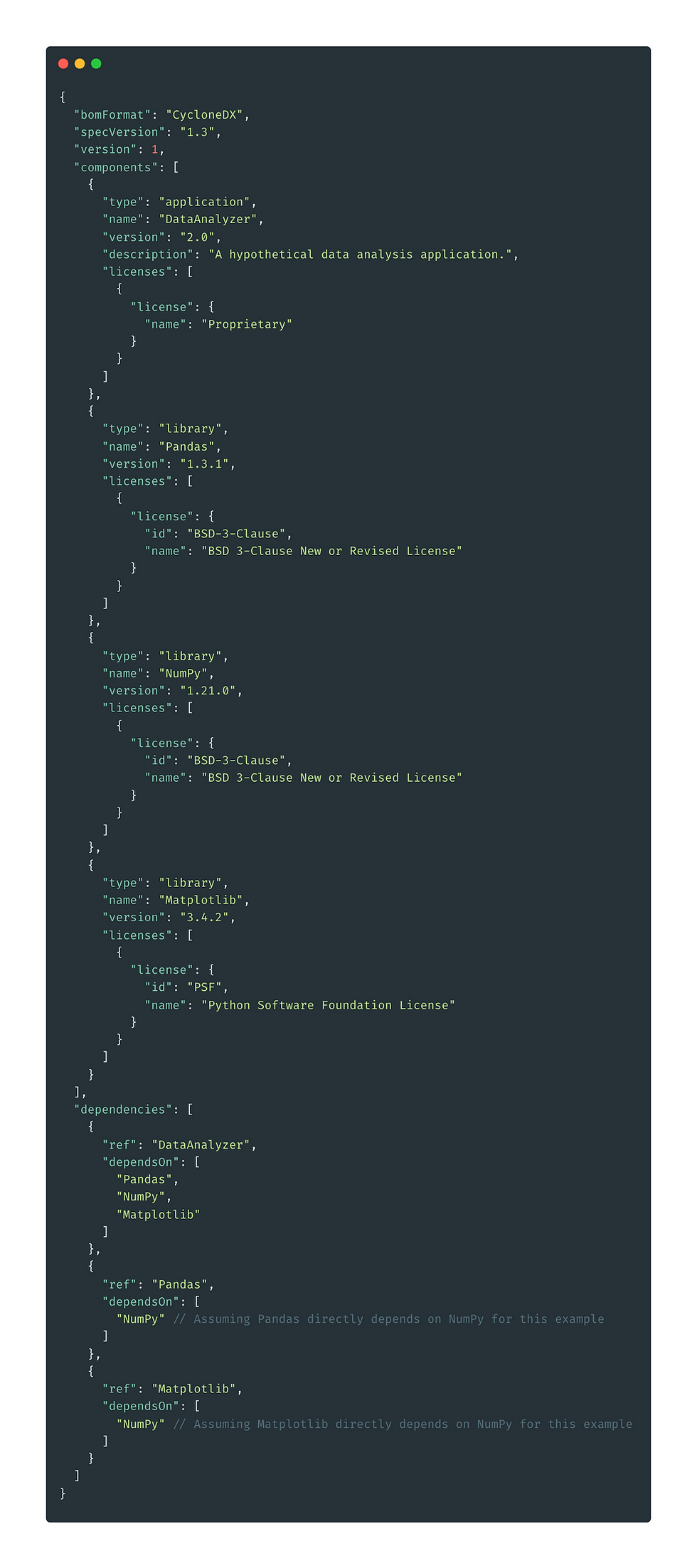
This JSON snippet outlines the core structure of a CycloneDX SBOM, highlighting each dependency's name, version, and license. It serves as a machine-readable document that can be easily integrated into software development and security analysis workflows.
SPDX Example for DataAnalyzer
SPDX (Software Package Data Exchange) is another prominent SBOM standard that facilitates the exchange of software package data. Below is an example of how the DataAnalyzer application's dependencies might be represented in an SPDX document:
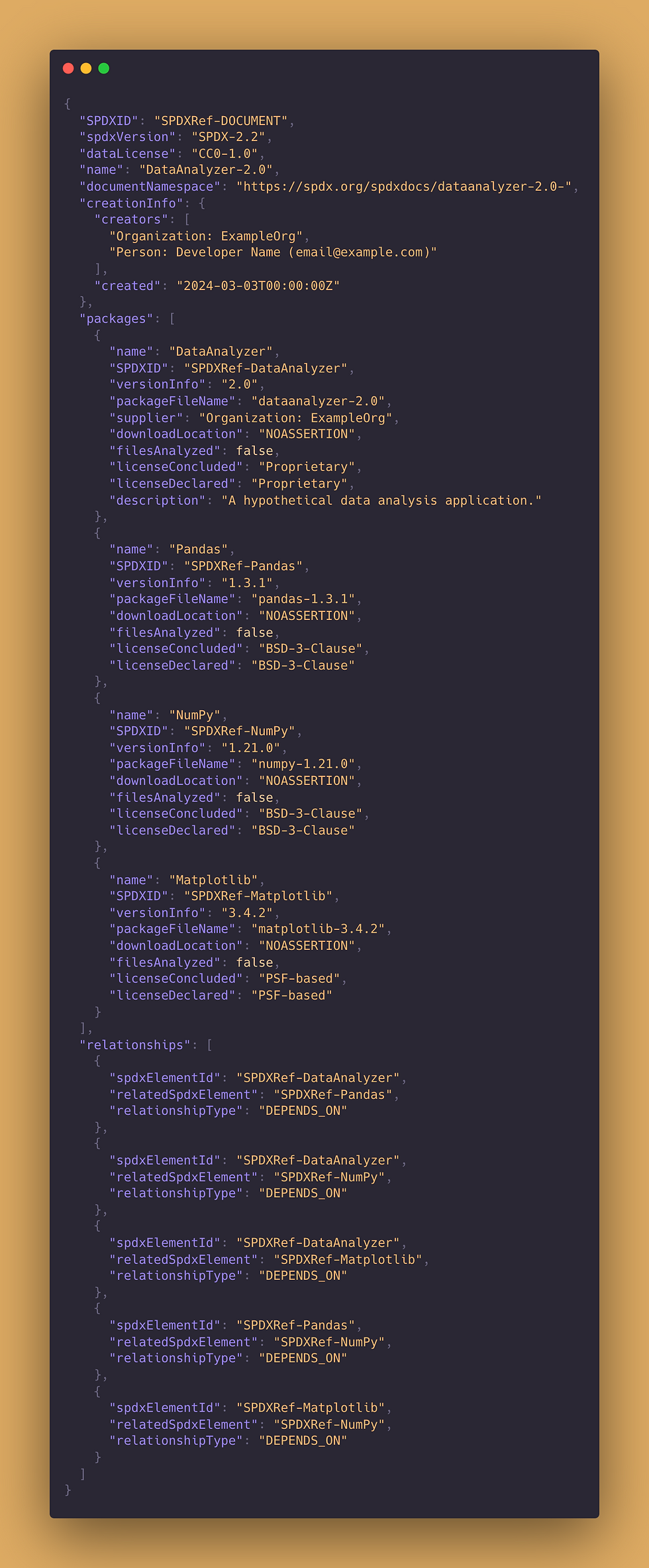
This document outlines the dependencies, versions, and licenses in a structured format, making it easy to track and analyze the software components and their compliance status.
SBOM Standard | Organization | Description | Format | License information | Vulnerability Data | Adoption | Additional Notes |
---|---|---|---|---|---|---|---|
SPDX (Software Package Data Exchange) | Linux Foundation | A standard to provide a common format for sharing data about software components and their associated licenses, copyrights, and security vulnerabilities. | XML, JSON, YAML, RDF, tag-value | ✅ | ✅(via external references) | Widely adopted in the open-source community. |
|
CycloneDX (Cyclone Dependency Exchange) | OWASP | Designed to provide a lightweight, extensible, and technology-agnostic method to create a software bill of materials for application security and supply chain transparency. | XML, JSON | ✅ | ✅(via external references) | Increasingly adopted, particularly in security-focused organizations. |
|
Static Analysis: The Foundation of SBOM Generation
At its core, static analysis involves examining software source code and binaries without executing them. This process is crucial for identifying both direct and indirect software dependencies and components. Imagine you're reading through a recipe without actually cooking it; you're trying to understand the ingredients and steps involved.
Tools like Snyk and Sonatype Nexus are champions in this arena. They sift through code and metadata, pinpointing connections between libraries, frameworks, and other software pieces.
Here's a simplified code snippet to illustrate static analysis:
# Example of static analysis in Python
import ast
def analyze_dependencies(code):
tree = ast.parse(code)
for node in ast.walk(tree):
if isinstance(node, ast.Import):
print(f"Imported module: {node.names[0].name}")
elif isinstance(node, ast.ImportFrom):
print(f"From {node.module} import {node.names[0].name}")
code_example = """
import requests
from os import path
"""
analyze_dependencies(code_example)
This Python example demonstrates a basic form of static analysis, identifying import statements to infer dependencies.
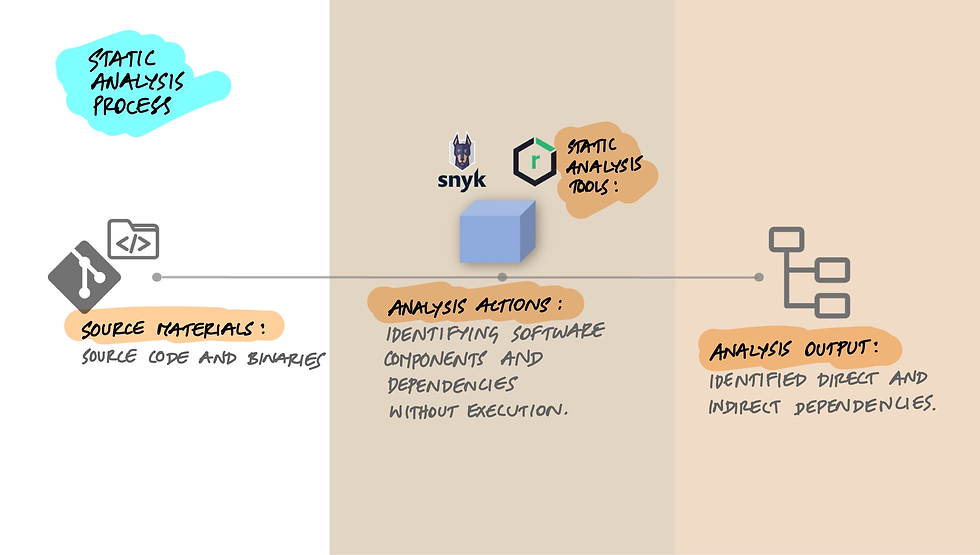
Dynamic Analysis: Observing Software in Motion
Dynamic analysis takes a different approach by running software in a controlled environment to observe its behavior, focusing on runtime operations and interactions. This method can uncover transitive dependencies, which are only visible when the software is in action. JFrog Xray is a prime example of a tool that excels in dynamic analysis, offering insights into the runtime behavior of applications.
Consider a scenario where your application dynamically loads plugins or modules based on user input or configuration. Dynamic analysis helps in mapping these runtime behaviors to understand the application's dependency tree fully.
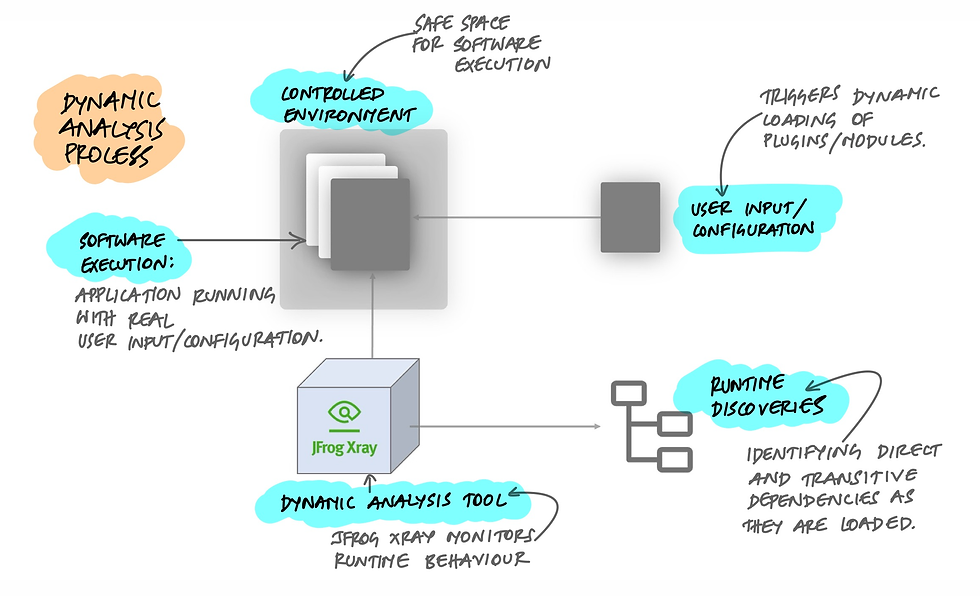
Package Managers: The Unsung Heroes
Package managers play a pivotal role in SBOM generation by automating dependency listing. Most programming languages come equipped with their own package managers, such as npm for Node.js and pip for Python. Here's how you can generate an SBOM using these package managers:
For Node.js projects:
npm ls --prod --json > sbom.json
For Python projects:
pip freeze > sbom.txt
These commands create an SBOM listing all the dependencies and their versions used in your project, providing a clear picture of your project's external software components.
SBOM Generation for Container Images
With the rise of containerized applications, tools like Trivy have become indispensable for generating SBOMs from container images. Trivy scans container images for vulnerabilities and generates a comprehensive list of OS-level packages and application-level dependencies.
Crafting a Container-Level SBOM with Trivy
Let's take a pass at the steps involved in generating the container level sbom using trivy
1. Pulling or Loading the Container Image
The first step involves acquiring the container image you intend to analyze. This can be done by pulling the image from a remote registry using commands like docker pull <image_name>, or by loading an image from a local file if you have it stored offline. This step ensures you have the exact version of the image you want to analyze available locally.
2. Extracting the Container Image
Container images are structured in layers, building upon a base image to form the final product. To analyze the content, you need to decompose the image into its constituent layers. Tools like Docker offer functionalities such as docker save and docker export to save these layers into a file system structure, making it possible to examine the contents of each layer individually.
3. Identifying the Operating System and Base Image
Understanding the base image and operating system of your container is crucial. This information provides context for the rest of the analysis, as it influences the types of packages and dependencies that might be present. Identifying the base OS helps tailor the analysis process to the specific package management systems and file structures of that environment.
4. Listing and Cataloging OS-Level Packages
With knowledge of the operating system, you can proceed to list all OS-level packages within the container's file system. Depending on the OS, you would use the appropriate package manager's list command, such as dpkg -l for Debian-based systems or rpm -qa for RedHat-based systems. This step compiles a list of installed packages along with their versions.
5. Detecting Application-Level Dependencies
Beyond OS-level packages, containers often include application-specific dependencies defined in files like package.json for Node.js, requirements.txt for Python, or Gemfile for Ruby. Language-specific tools can parse these files to list out the dependencies they define, offering a view into the application-level components of your container.
6. Scanning for Embedded Libraries
Some applications bundle embedded libraries within the container. Scanning the filesystem for known library file types, such as .jar files for Java or .so files for shared libraries in Linux, helps identify these embedded components. Tools like find or other scanning utilities can facilitate this search.
7. Analyzing Custom Code and Binaries
For custom code and binaries present in the container, static analysis tools can be employed to attempt to identify any dependencies or libraries they might be using. This analysis helps in cataloging not just the off-the-shelf components but also the custom elements unique to the container.
8. Documenting and Cataloging All Components
After identifying all components, the next step is to document and catalog each one, detailing the name, version, source/path, and other relevant information. This comprehensive list forms the core of your SBOM, offering a complete snapshot of the software components within your container.
9. Optional: Cross-Referencing with Known Databases
Optionally, you can enhance the SBOM by cross-referencing the identified components with known vulnerability or compliance databases. This adds valuable metadata, such as known vulnerabilities or licensing information, providing a deeper layer of insight into the security and compliance posture of the container.
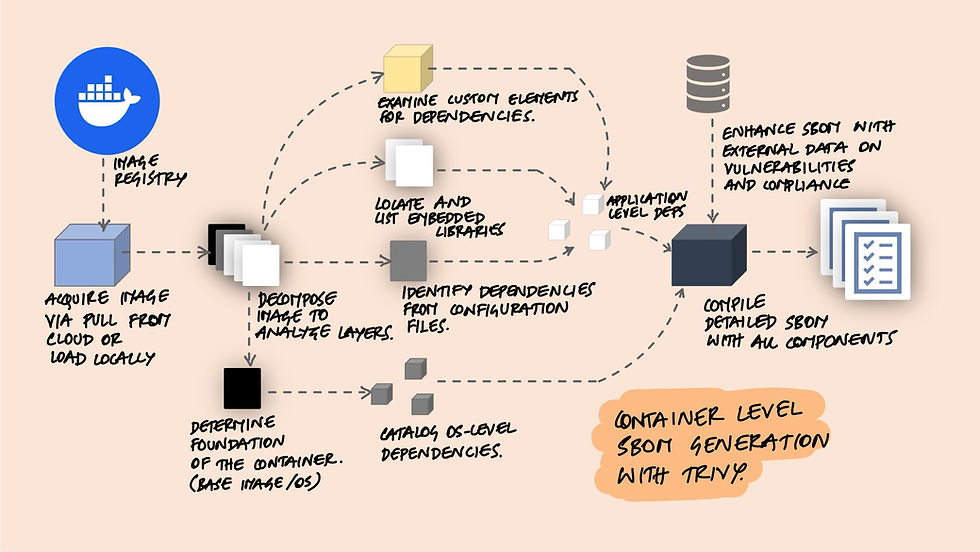
Navigating the Challenges of SBOM Generation
Now let’s take a look at the obstacles encountered in SBOM generation and analysis, highlighting the issues of data availability, tool maturity, and the identification of exploitable vulnerabilities.
The Hurdles of Data Availability and Vendor Customization
One of the first major roadblocks in SBOM generation is the inconsistent adherence to industry standards such as SPDX (Software Package Data Exchange) and CycloneDX. These standards aim to provide a uniform format for documenting the components of software packages, but they are not always fully implemented in practice.
Data Availability: Not all software vendors provide comprehensive data on their components, often due to the lack of readily available information. This gap in data can leave critical dependencies undocumented, obscuring potential security risks.
Vendor Customization: Some vendors choose to include only a subset of the data recommended by standards, or they may tailor these standards to fit their unique requirements. This practice has led to the emergence of non-standard SBOM formats, complicating the analysis and comparison of SBOMs across different software products.
"An incomplete SBOM is like a puzzle with missing pieces; you can guess the overall picture, but you'll never see the full image or understand where the vulnerabilities truly lie."
For instance, a vendor might release a proprietary software tool with an SBOM that omits certain dependencies due to proprietary concerns, or they might use a customized SBOM format that aligns with their internal processes but complicates interoperability with standard SBOM tools.
The Maturity Gap in SBOM Tools
SBOM tools are designed to automate the generation and analysis of SBOMs, but the landscape of these tools reveals significant variability in usability, standardization, and interoperability.
Lack of Usability: Many SBOM tools are complex and not user-friendly, posing challenges for teams without specialized expertise.
Inconsistency and Lack of Standardization: Tools may interpret standards differently, leading to variations in the SBOMs generated for the same software component across different tools.
Interoperability Issues: The lack of a common framework for SBOM formats means that tools may not work seamlessly together, hindering the exchange and comparison of SBOM data across different platforms.
An example could be two different SBOM generation tools analyzing the same application but producing different outputs due to differing interpretations of what constitutes a component or how to list dependencies, leading to confusion and inefficiency in vulnerability assessment.
Identifying and Communicating Exploitable Vulnerabilities
While SBOMs are invaluable for identifying software components and associated vulnerabilities, not all vulnerabilities present the same level of risk. The challenge lies in discerning which vulnerabilities are actually exploitable in a given context.
Vulnerability Exploitability Exchange (VEX) Documents: VEX documents are intended to provide clarity on the exploitability of vulnerabilities within specific environments. However, their effectiveness is hampered by reliability issues, as they rely on accurate and timely updates from vendors and security communities.
The need for more effective communication mechanisms is evident. For example, a software component may be listed as vulnerable in an SBOM, but without contextual information about its configuration or usage within the application, it's difficult to assess the actual risk. This lack of clarity can lead to unnecessary alarm or, conversely, complacency.
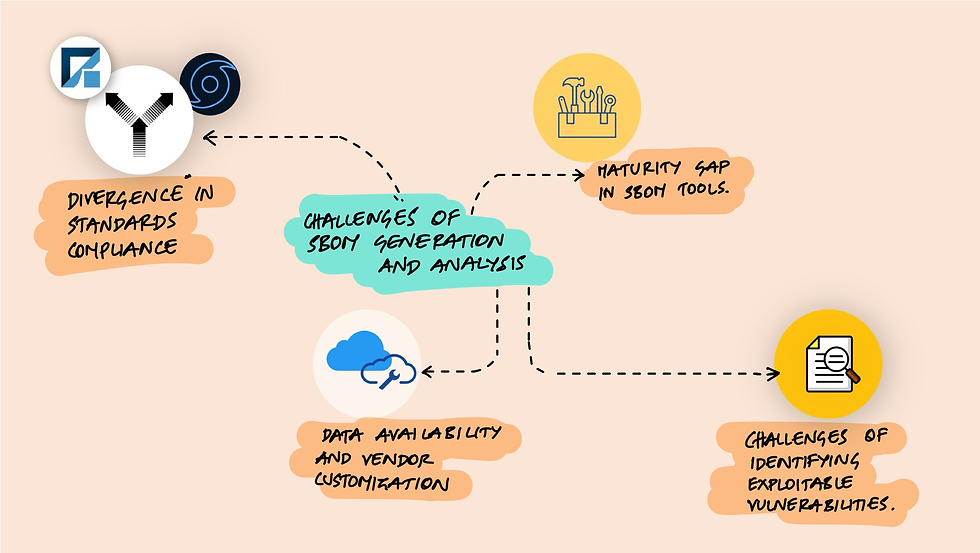
The Role of Software Composition Analysis (SCA) Tools
To manage and analyze SBOMs efficiently, developers turn to Software Composition Analysis (SCA) tools. These tools automate the process of scanning applications to identify all the components they use, generating an SBOM from the findings. Integrating SCA tools into your Continuous Integration (CI) pipeline is a game-changer, automating SBOM generation and analysis as a regular part of building software.
SBOM Analysis in the CI Pipeline
For SBOMs to truly serve their purpose, they must be comprehensive and accurate. An incomplete SBOM might actually do more harm than good by offering a false sense of security. It's critical that the chosen SCA tool is compatible with the project's programming language and package manager and that it identifies every dependency, direct or indirect. If no single tool covers all bases, you might need to employ multiple tools and then combine their outputs into a unified SBOM.
How to Implement
Here's a straightforward example of how you might integrate SBOM generation into a CI pipeline using a hypothetical SCA tool:
jobs:
sbom_generation:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Install SCA tool
run: sudo apt-get install sca-tool
- name: Generate SBOM
run: sca-tool generate --output sbom.json
- name: Merge SBOMs (if using multiple tools)
run: sca-tool merge sbom1.json sbom2.json --output merged_sbom.json
- name: Upload SBOM
uses: actions/upload-artifact@v2
with:
name: SBOM
path: merged_sbom.json
In this simplified pipeline, the software checks out the code, installs the SCA tool, generates an SBOM, optionally merges SBOMs from different tools, and then uploads the final SBOM as a build artifact.
Practical Tips for SBOM Integration
Choose the right tools: Ensure the SCA tools you select support your project's languages and package managers.
Automate within the CI pipeline: Integrate SBOM generation and analysis directly into your CI pipeline to ensure it's a seamless part of your development process.
Merge and manage SBOMs: If you use multiple tools to cover different aspects of your project, be prepared to merge their outputs into a single, comprehensive SBOM.
SBOM generation and analysis tools
Let's dive into some popular SBOM generation tools, understand their functionalities, and see how they fit into the development process.
Snyk
Snyk has carved out a niche for itself in the SBOM analysis ecosystem by leveraging API calls to its centralized database of packages and frameworks. This approach enables Snyk to offer detailed insights into your software components, making it a go-to tool for generating SBOMs, managing dependencies, and setting up alerts for vulnerabilities.
Snyk's platform is comprehensive, covering various aspects of software security and dependency management. However, while Snyk offers a generous freemium plan, some use cases may require the premium plan, potentially leading to a reliance on Snyk's ecosystem.
GitHub's Approach to SBOM and Security
GitHub offers a native solution for generating SBOMs through its dependency graph feature, accessible under Insights -> Dependency Graph. This feature primarily supports the SPDX format and relies on static code analysis to generate the SBOM. While there are concerns about the depth of information provided, GitHub compensates by allowing the submission of SBOMs from third-party tools.
Moreover, GitHub enhances its security offerings with Dependabot, an automated tool that monitors your dependencies for vulnerabilities and updates. Dependabot simplifies dependency management by automatically creating pull requests for updating to safer versions, thus streamlining the update process.
CycloneDX
CycloneDX stands out for its ability to generate SBOMs without external API calls, using static code analysis of your project's manifest and lock files. It is a CLI tool developed by core contributors of OWASP Dtrack. This tool is designed to support various programming languages and frameworks, making it a versatile choice for developers.
Key features of CycloneDX include:
SBOM Generation: It generates SBOMs directly from your project files.
Validation: CycloneDX validates the completeness and accuracy of the SBOMs, providing detailed logs for any issues detected.
Analysis: It can identify components with multiple versions in use, helping streamline dependency management.
Keygen and Verify: This feature adds a layer of security by allowing BOM signing and verification to protect against tampering.
Merge SBOMs: For projects using multiple tools or languages, CycloneDX can merge several SBOMs into a single document.
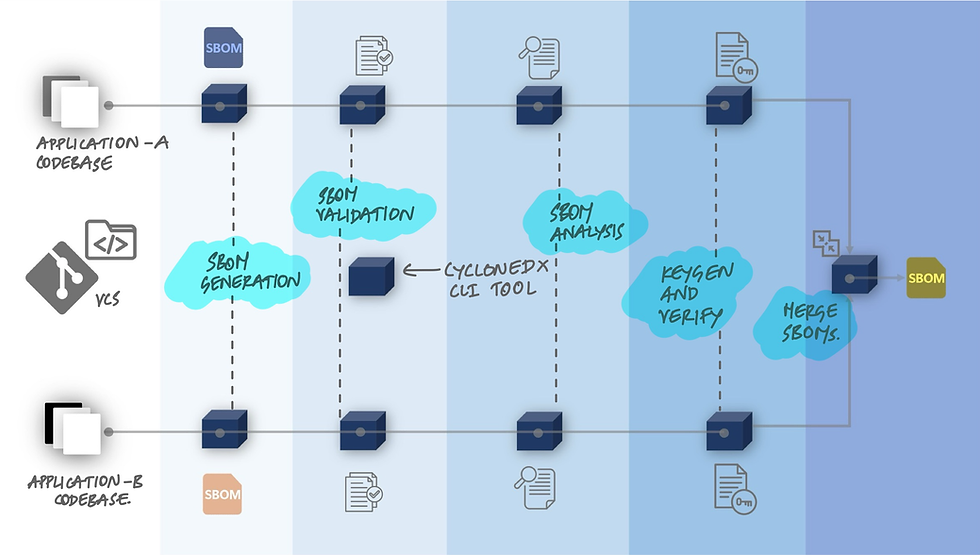
OWASP Dependency-Track
Dependency-Track, an open-source tool developed under the OWASP umbrella, has gained popularity for its comprehensive approach to SBOM analysis. Since its inception in 2013, Dependency-Track has evolved from a simple inventory tracker to a sophisticated tool used by thousands of organizations worldwide.
Dependency-Track emphasizes continuous SBOM analysis, offering features for security, license compliance, and risk management. Its integration capabilities make it ideal for CI/CD workflows, allowing developers to maintain high standards of security and compliance without sacrificing speed or efficiency.
How Dependency-Track Fits into DevOps
Seamless integration into Continuous Integration (CI) and Continuous Deployment (CD) pipelines is a foundational aspect of Dependency-Track's design. It offers a robust API and supports plugins for popular CI tools like Jenkins, making it a DevOps-friendly solution. This integration allows development teams to automate the generation and analysis of SBOMs, ensuring that every build is checked for vulnerabilities and compliance issues.
Key Features of Dependency-Track
Dependency-Track stands out by offering a wide array of features:
Vulnerability Detection: Automatically identifies security vulnerabilities in components.
Policy Evaluation: Checks components against predefined security and compliance policies.
Impact Analysis: Assesses the potential impact of vulnerabilities on the application.
Exploit Prediction: Predicts the likelihood of a vulnerability being exploited.
Auditing Workflow: Streamlines the process of reviewing and addressing vulnerabilities.
Outdated Version Detection: Flags the use of outdated software components.
Full-Stack Inventory: Provides a complete overview of all software components, from the application layer down to the operating system.
Bill of Materials (BOM) Analysis: Analyzes the composition of your software for better insight and control.
Vulnerability Aggregation: Consolidates vulnerability data from multiple sources for comprehensive analysis.
Notifications and Integrations: Supports alerts and integrates with other tools to enhance the development workflow.
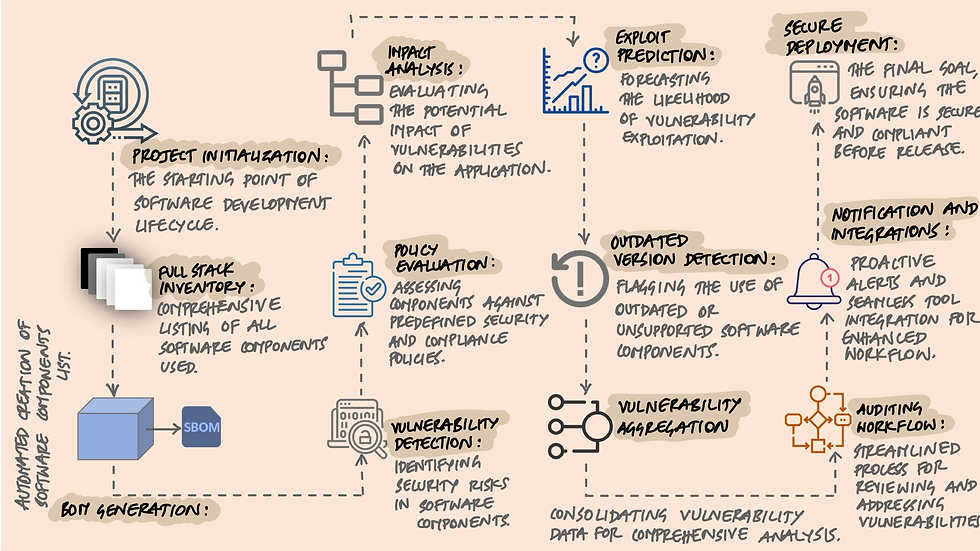
Implementing Dependency-Track in Your CI/CD with GitHub Actions
Integrating Dependency-Track with GitHub Actions can significantly enhance your CI/CD pipeline by automating the generation, upload, and analysis of SBOMs. Here's an example workflow that demonstrates how you can use CycloneDX to generate an SBOM, upload it to Dependency-Track, and then retrieve feedback on any policy violations directly in GitHub Actions:
name: SBOM Analysis with Dependency-Track
on: [push]
jobs:
sbom-analysis:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Generate SBOM with CycloneDX
run: |
# Install CycloneDX
npm install -g @cyclonedx/bom
# Generate SBOM
cyclonedx-bom -o bom.xml
- name: Upload SBOM to Dependency-Track
uses: actions/upload-artifact@v2
with:
name: SBOM
path: bom.xml
- name: Analyze SBOM in Dependency-Track
run: |
# This step would involve calling Dependency-Track's API to upload the SBOM
# and then polling for the status of the analysis. You'll need to replace
# this with actual API calls and handling based on Dependency-Track's API documentation.
echo "Upload and analyze SBOM in Dependency-Track"
- name: Check for Policy Violations
run: |
# After analysis, fetch any policy violations from Dependency-Track
# This would also involve API calls to Dependency-Track to retrieve the results
echo "Retrieve policy violation feedback from Dependency-Track"
This workflow showcases a simplified process where you generate an SBOM using CycloneDX, upload it for analysis in Dependency-Track, and then check for any policy violations. It's a powerful example of how automated tools can streamline the identification and management of software vulnerabilities in a CI/CD pipeline.
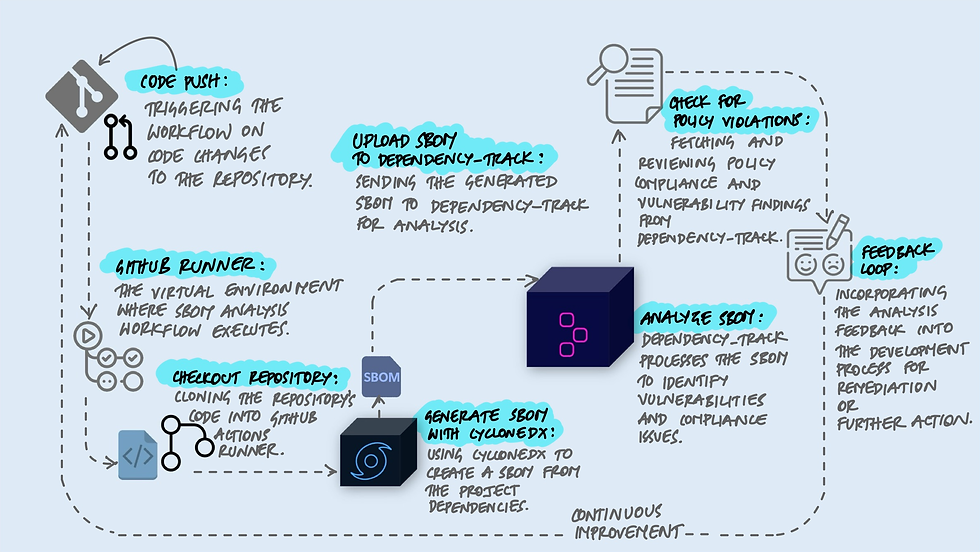
Setting Up Dependency-Track with Helm
Helm, the package manager for Kubernetes, simplifies the deployment of apps like Dependency-Track. To install Dependency-Track using Helm, you'd typically follow these steps:
Add the Helm Repository: First, you need to add the repository that contains the Dependency-Track chart. In our example, we use a chart maintained by DavidKarlsen.
helm repo add evryfs-oss <https://evryfs.github.io/helm-charts/>
Install Dependency-Track:With the repository added, you can now install Dependency-Track using the Helm chart. Note the version number might change, so always check for the latest version.
helm install my-dependency-track evryfs-oss/dependency-track --version 1.3.5
This command deploys Dependency-Track on your Kubernetes cluster, ready for you to start uploading and analyzing SBOMs.
Dependency-Track: Features and Interfaces
Once installed, Dependency-Track offers a rich set of features and a user-friendly interface for managing software vulnerabilities and compliance.
Dashboard Overview
The project's dashboard is your command center, presenting a comprehensive view of your project's security posture. Here, you can quickly access summaries of vulnerabilities, policy violations, and other critical metrics at a glance.
Understanding the Dependency Graph
A core feature of Dependency-Track is its dependency graph. This visual tool helps in Root Cause Analysis (RCA), impact analysis, and understanding transitive dependencies across a wide range of components including applications, libraries, frameworks, and more. It's an invaluable asset for identifying how vulnerabilities in one component might affect others.
Auditing Vulnerabilities
Dependency-Track stores SBOMs in a PostgresDB, allowing for efficient querying and management. Users can audit vulnerabilities directly from the interface, leveraging features like license grouping and policy creation to manage compliance and risk. For instance, you can create policies to suppress false-positive vulnerabilities or to enforce compliance with specific licensing requirements.
Exploit Predictions: CVSS and EPSS
When facing a multitude of identified vulnerabilities, prioritizing them becomes crucial. Dependency-Track's exploit prediction feature utilizes two key metrics: the Common Vulnerability Scoring System (CVSS) and the Exploit Prediction Scoring System (EPSS).
CVSS provides a standardized way to rate the severity of vulnerabilities based on factors like attack vector and complexity.
EPSS offers a probability score reflecting the likelihood of a vulnerability being exploited, taking into account historical data on similar vulnerabilities.
Together, these metrics guide you in prioritizing vulnerabilities for mitigation, focusing your efforts where they are most needed.
Prioritizing Vulnerabilities
Imagine a scenario where you have two vulnerabilities in your system. One has a high CVSS score indicating severe impact, but a low EPSS score suggesting it's unlikely to be exploited soon. The other has a moderate CVSS score but a high EPSS score. Dependency-Track helps you prioritize the latter for immediate action, ensuring you tackle the most pressing threats first.
In wrapping up our series on the significance of Software Bill of Materials (SBOM) generation and analysis, it's clear that as the complexity of software development escalates, the necessity for transparency and security through SBOMs becomes undeniable. Tools like CycloneDX and Dependency-Track, along with automation in CI/CD pipelines, mark a pivotal shift towards integrating comprehensive, automated SBOM practices into the software development lifecycle.
コメント