Disposability in Software Development
- Visakh Unni
- Jan 29
- 2 min read
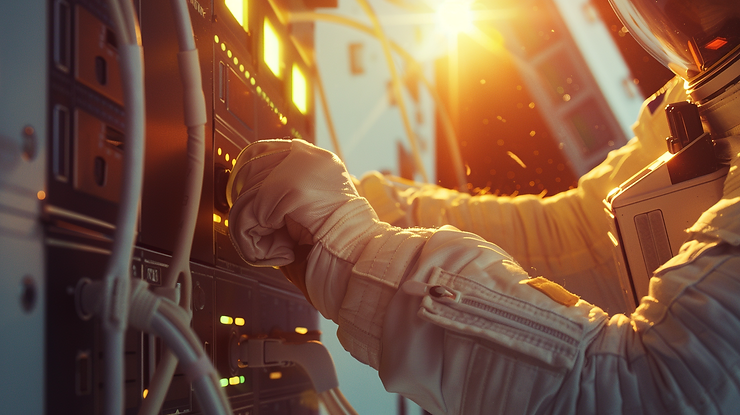
Embrace Efficiency: Swift Startup and Graceful Shutdown for Robust Applications
Having already mastered the art of developing functional applications, let's now turn our focus towards enhancing their resilience and flexibility. This brings us to the concept of disposability in the context of 12-factor apps—a methodology that emphasizes the importance of applications being able to start quickly and shut down gracefully. This approach is key to achieving robustness, facilitating rapid scaling, and ensuring smooth deployment processes.
"For a system to exhibit true robustness, it must achieve rapid activation upon initialization. However, robustness extends beyond mere startup; it encompasses efficient termination processes as well. It is crucial to engineer a system that can gracefully decommission, thereby safeguarding its integrity for subsequent operational cycles."
Fast Startup: The Sprinter's Approach to Launching Processes
The essence of fast startup in 12-factor apps lies in minimizing the time it takes for processes to move from initiation to operational state. This agility is crucial for quick releases and scaling operations. Imagine deploying a new feature or service; you want this process to be as swift as a sprinter off the blocks. This not only enhances the user experience by reducing downtime but also plays a pivotal role in operational efficiency.
Achieving Fast Startup
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
The fast startup in this Python Flask example is achieved through its minimal and straightforward setup, allowing it to serve HTTP requests immediately without complex configurations or external dependencies.
Graceful Shutdown: The Art of a Well-Planned Exit
Graceful shutdown is about ending processes without causing disruption. This involves ceasing to accept new requests, completing ongoing tasks, and then safely exiting. For web applications, this means finishing current HTTP requests. For worker processes, it involves tasks like returning the current job to the queue to ensure no work is lost.
Implementing Graceful Shutdown
import signal
import time
def graceful_shutdown(signum, frame):
print("Shutting down gracefully...")
# Perform cleanup tasks here
# For example, save state, complete transactions, etc.
time.sleep(1) # Simulate cleanup task
print("Shutdown complete.")
exit(0)
signal.signal(signal.SIGTERM, graceful_shutdown)
print("Application started. Press Ctrl+C to exit.")
while True:
time.sleep(1)
This Python script demonstrates handling a SIGTERM signal to perform a graceful shutdown, ensuring that the application cleans up resources and exits cleanly.
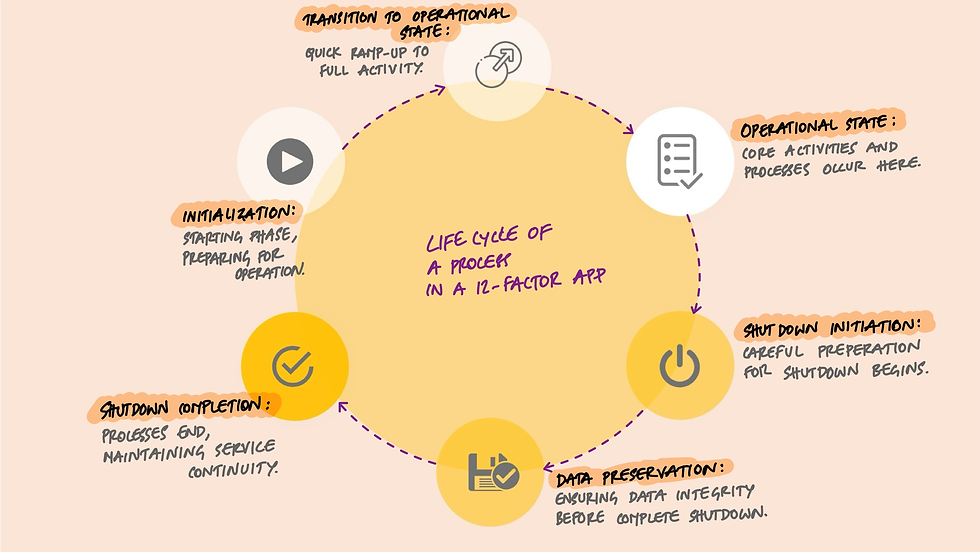
Commentaires